Real Scenario-Based Questions on Lightning Web Components (LWC)
In the dynamic world of Salesforce development, mastering Lightning Web Components (LWC) is essential for creating efficient and responsive user interfaces. As businesses increasingly rely on Salesforce to streamline their processes, developers with expertise in LWC are in high demand. To prepare for the challenges that come with real-world projects and interviews, it's crucial to have a solid grasp of LWC's capabilities in practical scenarios.
Asking simple straightforward questions in Lightning Web Components (LWC) is history. Few years back Salesforce certified people were very less. Hence criteria was just to check whether that person know about basic Lightning Web Component (LWC) or not.
So questions used to be:
• What is Lightning Web Components (LWC)?
• How is LWC different from Aura?
• What is the use of a Lightning Component Framework?
• What does a component bundle contain?
• Explain the concept of data binding in LWC?
• What is the use of the Salesforce Lightning Design System?
etc.
If you notice above questions are crisp to the point. But now since in market there are many Salesforce certified people above style of interview doesn't work. One could easily memories these.
I too take interviews in my company and now style is different. Focus is more to ask scenario questions rather then what how etc.
This blog delves into a comprehensive collection of `real scenario-based questions on Lightning Web Components`, offering valuable insights and hands-on examples. These questions are designed to simulate real-world situations that developers often encounter during application development, making them an excellent resource for both beginners and experienced developers looking to enhance their skills.
Each scenario is tailored to focus on specific aspects of LWC development, ranging from the basics to more advanced topics. The questions touch upon various areas, including:
1. Component Communication and Interaction: Learn how to effectively communicate between components using properties and events, utilizing event bubbling and composing to share data seamlessly.
2. Integration with External Libraries: Explore the world of third-party JavaScript libraries and how to integrate them smoothly into your LWC components using the "loadScript" utility.
3. Dynamic Styling and Responsive Design: Discover how to apply dynamic styles and create responsive designs that adapt to different screen sizes based on user input.
4. Data Management and Apex Integration: Understand data management within LWC by utilizing reactive properties and the "wire" service. Learn how to interact with Apex classes to fetch and manipulate Salesforce data.
5. Interactive UI Elements: Implement custom notifications and create interactive charts, searchable components, and multi-step wizards to enhance user interaction.
6. Real-Time Updates and Location Services: Build components that harness real-time updates through Lightning Message Service and utilize browser geolocation APIs to display user coordinates.
MIND IT !
Each scenario is accompanied by example code that demonstrates how to tackle the challenges presented. By practicing these real scenario-based questions, developers can gain a deeper understanding of LWC's functionalities and become better equipped to handle diverse development scenarios.
In a nutshell, this blog offers a user-friendly exploration of real scenario-based questions that shed light on the various dimensions of Lightning Web Components. Whether you're preparing for interviews or aiming to boost your LWC skills, these real-world scenarios and solutions provide a solid framework for becoming a proficient LWC developer.
Let start the interview series on `Real Scenario Based Interview Questions on Lightning Web Components Part -1` (Between Interviewer & Candidate).
In this blog series, I have tried to cover all `LWC Scenario Based Interview Questions` which are often asked by Salesforce Developer in an interview.
LWC Scenario 1 : Dynamic Form Creation
Question: You need to create a dynamic form with input fields that change based on user selection. How would you implement this in LWC?
Answer: Implementing a dynamic form with input fields that adapt based on user selection in Lightning Web Components (LWC) involves a combination of conditional rendering and event handling. Here's how you could approach it:
Step 1: Define the Component
Create a Lightning Web Component to house the dynamic form. Let's call it `DynamicForm`.
Step 2: Define the Form Fields
In the `DynamicForm` component, define the possible input fields that can be displayed based on user selection. For example, you can include fields for name, email, phone number, and address.
<template> <lightning-combobox label="Select Form Type" value={selectedFormType} options={formTypeOptions} onchange={handleFormTypeChange}></lightning-combobox> <template if:true={showNameField}> <lightning-input label="Name"></lightning-input> </template> <template if:true={showEmailField}> <lightning-input label="Email"></lightning-input> </template> </template>
Step 3: Handle User Selection
Create methods in the `DynamicForm` component's JavaScript file to handle user selections and toggle the visibility of input fields accordingly.
// JS File - dynamicForm.js import { LightningElement, track } from 'lwc'; export default class DynamicForm extends LightningElement { @track selectedFormType = ''; @track showNameField = false; @track showEmailField = false; // Add similar tracking variables for other fields formTypeOptions = [ { label: 'Select Form Type', value: '' }, { label: 'Name', value: 'name' }, { label: 'Email', value: 'email' }, // Add options for other fields ]; handleFormTypeChange(event) { this.selectedFormType = event.detail.value; this.showNameField = this.selectedFormType === 'name'; this.showEmailField = this.selectedFormType === 'email'; // Update similar variables for other fields } }
Step 4: Display the Dynamic Form
Include the `DynamicForm` component in a parent component or app where the user can interact with it.
Output:
In this setup, the user can choose a form type from a dropdown, and the corresponding input fields will dynamically appear based on their selection. This approach allows you to create flexible and adaptive forms in LWC that respond to user preferences.
LWC Scenario 2 : Display Records with "wire" Service
Question: Fetch a list of Account records using the "wire" service and display them in a Lightning Web Component.
Answer: To fetch and display a list of account records using the "wire" service in Lightning Web Components (LWC), you can use the `wire` decorator along with the `getRecord` or `getListUi` adapter. Here's how you can achieve this:
Step 1: Define the Component.
Create a Lightning Web Component to display the list of account records. Let's call it `AccountList`.
Step 2: Fetch and Display Account Records
In the `AccountList` component's JavaScript file, use the `wire` decorator to fetch the account records using the `getListUi` adapter. The `getListUi` adapter allows you to retrieve a list of records and their associated data. Here's how you can do it:
// JS File - accountList.js import { LightningElement, wire } from 'lwc'; import { getListUi } from 'lightning/uiListApi'; import ACCOUNT_OBJECT from '@salesforce/schema/Account'; export default class AccountList extends LightningElement { @wire(getListUi, { objectApiName: ACCOUNT_OBJECT, listViewApiName: 'AllAccounts' }) accounts; get accountList() { return this.accounts.data ? this.accounts.data.records.records : []; } }
In this example, the `getListUi` adapter is used to fetch account records from the standard "AllAccounts" list view. The `accounts` property is automatically populated with the retrieved data.
Step 3: Display Account Records
In the `AccountList` component's HTML file, use iteration to display the fetched account records:
- {account.fields.Name.value}
In this example, the fetched account records are iterated over and displayed in a list format.
Output:
By following these steps, you're effectively using the "wire" service with the getListUi adapter to fetch and display a list of account records in a Lightning Web Component.
LWC Scenario 3 : Dynamic Form Creation
Question: When a user submits a record, handle any errors and provide feedback using toasts.
Answer:
HTML (submitRecord.html):
<lightning-input label="Name" value={recordName} onchange={handleNameChange}></lightning-input> <lightning-button label="Submit" onclick={handleSubmit}></lightning-button>
JavaScript (submitRecord.js):
// JS File - submitRecord.js import { LightningElement, track } from 'lwc'; import { ShowToastEvent } from 'lightning/platformShowToastEvent'; export default class SubmitRecord extends LightningElement { @track recordName = ''; handleNameChange(event) { this.recordName = event.target.value; } handleSubmit() { if (this.recordName) { // Simulate record submission and handle success this.handleSuccess(); } else { // Handle error and show toast this.handleError('Please enter a valid name.'); } } handleSuccess() { // Handle successful submission and show success toast const event = new ShowToastEvent({ title: 'Success', message: 'Record submitted successfully.', variant: 'success', }); this.dispatchEvent(event); } handleError(errorMessage) { // Handle error and show error toast const event = new ShowToastEvent({ title: 'Error', message: errorMessage, variant: 'error', }); this.dispatchEvent(event); } }
In this example, when a user enters a name and clicks the "Submit" button, the `handleSubmit` method checks if a valid name is provided. If a valid name is entered, the `handleSuccess` method is called to show a success toast using the `lightning/platformShowToastEvent` module. If no name is provided, the `handleError` method is called to show an error toast.
Output:
showSuccessToast : If a valid name is entered, the `handleSuccess` method is called to show a success toast.
showErrorToast: If no name is provided, the `handleError` method is called to show an error toast.
The `lightning/platformShowToastEvent` module provides an easy way to display toast notifications in Lightning Web Components. You can customize the appearance and behavior of the toast using the properties of the `ShowToastEvent` object.
MIND IT !
Remember to include the necessary Lightning Design System styles in your component for proper styling. You can also enhance this example by integrating it with actual record submission logic and handling more complex scenarios.
LWC Scenario 4 : Component Communication
Question: Implement communication between two sibling components via a shared parent component.
Answer: Implementing communication between two sibling components via a shared parent component is a common requirement in Lightning Web Components (LWC). This approach allows you to pass data or trigger actions between components that are not directly connected. Here's how you can achieve this:
Step 1: Create the Parent Component
Create a parent component that will serve as the mediator between the two sibling components. Let's call it ParentComponent.
Step 2: Define Sibling Components
Create two sibling components that need to communicate with each other. Let's call them `SiblingComponentA` and `SiblingComponentB`.
Step 3: Communication Flow
Here's how the communication will flow:
1. `SiblingComponentA` will send data to the `ParentComponent`.
2. The `ParentComponent` will receive the data and pass it to `SiblingComponentB`.
Step 4: Define the Parent Component
In the HTML file of `ParentComponent`, include the two sibling components and define a method to receive and pass data.
In the JavaScript file of `ParentComponent`, define the method to handle data changes and pass the data between the sibling components.
// ParentComponent.js import { LightningElement, track } from 'lwc'; export default class ParentComponent extends LightningElement { @track sharedData = ''; handleDataChange(event) { this.sharedData = event.detail; } }
Step 5: Define Sibling Component A
In the HTML file of `SiblingComponentA`, create an input field to update the shared data and dispatch a custom event to notify the parent component.
In the JavaScript file of `SiblingComponentA`, define the method to handle input changes and dispatch a custom event to notify the parent component.
// SiblingComponentA.js import { LightningElement } from 'lwc'; export default class SiblingComponentA extends LightningElement { localData = ''; handleInputChange(event) { this.localData = event.target.value; const dataChangeEvent = new CustomEvent('datachange', { detail: this.localData }); this.dispatchEvent(dataChangeEvent); } }
Step 6: Define Sibling Component B
In the HTML file of `SiblingComponentB`, display the shared data received from the parent component.<
Shared Data: {data}
In the JavaScript file of `SiblingComponentB`, define a property to receive the shared data from the parent component.
// SiblingComponentB.js import { LightningElement, api } from 'lwc'; export default class SiblingComponentB extends LightningElement { @api data; }
By following these steps, you'll establish communication between two sibling components via a shared parent component. SiblingComponentA sends data to the ParentComponent, and then the ParentComponent passes that data to SiblingComponentB, allowing the two siblings to communicate effectively.
LWC Scenario 5 : Third-Party Library Integration
Question: Integrate the "Chart.js" library to create a bar chart in a Lightning Web Component.
Answer:"Chart.js" is a popular JavaScript library for creating interactive charts. We'll create an LWC that displays a bar chart using sample data.
Step 1: Create a New Lightning Web Component
Create a Lightning Web Component to display the Chart Bar. Let's call it `ChartComponent`.
Step 2: Add the "Chart.js" Library to Static Resources
1. Download the "Chart.js" library from the official website: https://www.chartjs.org/
2. Create a static resource in Salesforce with the downloaded "Chart.js" library files.
Step 3: Include the "Chart.js" Library in the Component
In the JavaScript file (chartComponent.js) of your component, import the "Chart.js" library from the static resource and create the bar chart:
// JS File - chartComponent.js import { LightningElement } from 'lwc'; import { loadScript, loadStyle } from 'lightning/platformResourceLoader'; import CHARTJS from '@salesforce/resourceUrl/ChartJs'; import { ShowToastEvent } from 'lightning/platformShowToastEvent'; export default class ChartComponent extends LightningElement { chartJsInitialized = false; renderedCallback() { if (this.chartJsInitialized) { return; } this.chartJsInitialized = true; loadScript(this, CHARTJS) .then(() => { this.initializeChart(); }) .catch(error => { this.dispatchEvent(new ShowToastEvent({ title: 'Error', message: 'Error loading Chart.js library', variant: 'error' })); }); } initializeChart() { const ctx = this.template.querySelector('canvas.donut').getContext('2d'); new window.Chart(ctx, { type: 'bar', data: { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Sales', data: [50, 30, 60, 40, 70], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } }); } }
In this example, we integrated the "Chart.js" library into an LWC named `ChartComponent` using the `loadScript` and `loadStyle` methods. The JavaScript file loads the "Chart.js" library from a static resource and initializes the bar chart using the `renderedCallback` lifecycle hook. By avoiding the CDN, you have more control over the library's version and can comply with security requirements.
Output:
Customize the static resource names and paths based on your setup. This approach allows you to seamlessly integrate "Chart.js" or other third-party libraries into your Lightning Web Components while managing the resources within your Salesforce environment.
LWC Scenario 6 : Custom Record Display
Question: Create a Lightning Web Component that fetches and displays a specific record based on user input.
Answer:
Candidate's Response:
To achieve this functionality, I would create a Lightning Web Component that allows users to input a record Id. Upon input, the component will use the `lightning-record-form` component to fetch and display the corresponding record's details. Let me walk you through the steps and provide an example code.
Step 1: Create a New Lightning Web Component
First, I'll create a new Lightning Web Component named `RecordFetcher`.
Step 2: Include the lightning-record-form Component
In the HTML file (`recordFetcher.html`) of my component, I will use the `lightning-record-form` component to fetch and display the record. I'll also define an input field to allow users to input the record's Id.
Fetch and Display Record
<lightning-input label="Record Id" value={recordId} onchange={handleRecordIdChange}> <lightning-record-form record-id={recordId} columns="2" object-api-name="Account" layout-type="Full" mode="view"> </lightning-record-form>
Step 3: Create the JavaScript Logic
In the JavaScript file (`recordFetcher.js`) of my component, I will implement the logic to handle user input and update the displayed record.
// JS File - chartComponent.js import { LightningElement, track } from 'lwc'; export default class RecordFetcher extends LightningElement { @track recordId = ''; handleRecordIdChange(event) { this.recordId = event.target.value; } }
Step 4: Include the Component
Finally, I'll include my `RecordFetcher` component in a parent component or app to visualize the fetched and displayed record.
With this approach, users will be able to input a record Id, and the component will use the `lightning-record-form` to fetch and display the corresponding record's details.
Output:
Interviewer's Possible Follow-up:
Interviewer: "Could you explain how you would handle scenarios where the record doesn't exist or an error occurs during the fetching process?"
Candidate: "I would incorporate error handling logic within the JavaScript code. If an error occurs during fetching, I could display a user-friendly error message using a toast or an alert."
By providing a detailed step-by-step explanation and an example code, the candidate demonstrates their understanding of creating a Lightning Web Component that fetches and displays a specific record based on user input. They also address potential follow-up questions regarding error handling, showcasing a comprehensive understanding of the concept.
MIND IT !
During an interview, when the candidate is asked to create a Lightning Web Component that fetches and displays a specific record based on user input, the interviewer is likely evaluating the candidate's understanding of key concepts related to Lightning Web Components (LWC), as well as their ability to effectively implement the required functionality. Here's what the interviewer might check for:
1. Understanding of LWC Basics: The interviewer will assess whether the candidate understands the fundamental concepts of Lightning Web Components, including how components are structured, how they interact with the Salesforce platform, and their role in the Lightning Experience.
2. Usage of Core Components: The candidate's familiarity with using core Lightning Web Components, such as `lightning-input` and `lightning-record-form`, will be observed. The candidate should be able to correctly implement these components to create a functional user interface.
3. User Input Handling: The interviewer will examine how the candidate handles user input. This includes whether the candidate correctly uses event handling mechanisms like `onchange` to capture and respond to user input.
4. Integration with Salesforce Data: The interviewer will evaluate the candidate's ability to integrate the Lightning Web Component with Salesforce data. This involves correctly utilizing the `lightning-record-form` component to fetch and display records based on user input.
5. Component Reusability: The interviewer may inquire about the candidate's approach to creating reusable components. This could involve discussions on parameterization, modularity, and adhering to best practices for creating maintainable components.
6. Error Handling: The interviewer might ask how the candidate plans to handle scenarios where the record does not exist or where an error occurs during the fetching process. An understanding of error handling mechanisms and providing user-friendly feedback will be assessed.
7. Coding Standards and Best Practices: The candidate's adherence to coding conventions, style guidelines, and best practices for LWC development will be observed. Clean and well-organized code is usually preferred.
8. Component Interaction: Depending on the scope of the question, the interviewer may also ask about how the newly created component could interact with other components or services within the Salesforce ecosystem.
9. Clarity in Communication: Beyond the technical implementation, the interviewer may evaluate the candidate's ability to clearly explain their thought process, solution, and the decisions they made during the implementation.
10. Problem Solving: If the candidate encounters any roadblocks or challenges during the implementation, the interviewer will gauge their problem-solving skills, ability to debug, and find appropriate solutions.
Overall, the interviewer is looking for a holistic understanding of Lightning Web Components, practical application of Salesforce development concepts, and the candidate's ability to create functional and user-friendly solutions using LWC.
LWC Scenario 7 : Data Filtering and Sorting
Question: Build a Lightning Web Component that displays a list of Contacts and allows users to filter and sort them.
Answer:
Candidate's Response:
Step 1: Create Lightning Web Component
First, I would create a new Lightning Web Component named "ContactList".
Step 2: Design the User Interface (contactList.html)
I'd design the UI to include input fields for filtering and a combobox for sorting. Here's the HTML code:
<lightning-input type="text" label="Filter Contacts" onchange={handleFilterChange}></lightning-input> <lightning-combobox label="Sort By" options={sortOptions} onchange={handleSortChange}></lightning-combobox> <template for:each={filteredContacts} for:item="contact"> <p key={contact.Id}>{contact.Name}</p> </template>
Step 3: Implement JavaScript Logic (contactList.js)
I'd define the JavaScript logic to handle filtering and sorting. Here's the JavaScript code:
// JS File - contactList.js import { LightningElement, wire } from 'lwc'; import getContacts from '@salesforce/apex/ContactController.getContacts'; export default class ContactList extends LightningElement { filterText = ''; sortField = ''; @wire(getContacts) contacts; get filteredContacts() { return this.contacts.data ? this.contacts.data.filter(contact => contact.Name.toLowerCase().includes(this.filterText.toLowerCase())) : []; } get sortOptions() { return [ { label: 'Name', value: 'Name' }, { label: 'Email', value: 'Email' } ]; } handleFilterChange(event) { this.filterText = event.target.value; } handleSortChange(event) { this.sortField = event.target.value; if (this.sortField) { this.contacts.data.sort((a, b) => (a[this.sortField] > b[this.sortField] ? 1 : -1)); } } }
Step 4: Create Apex Controller (ContactController.cls)
I'd create an Apex class to fetch Contact records from Salesforce:
public with sharing class ContactController { @AuraEnabled(cacheable=true) public static List<contact> getContacts() { return [SELECT Id, Name, Email FROM Contact]; } }
Step 5: Add Styling (contactList.css)
Lastly, I'd apply some CSS to style the component:
/* CSS File - contactList.css */ .slds-m-around_medium { margin: 20px; }
Output:
Data Filtering and Sorting By Name (Before)
Data Filtering and Sorting By Name (After) :
Here i am filtering and sorting the name those start from the `J` alphabet
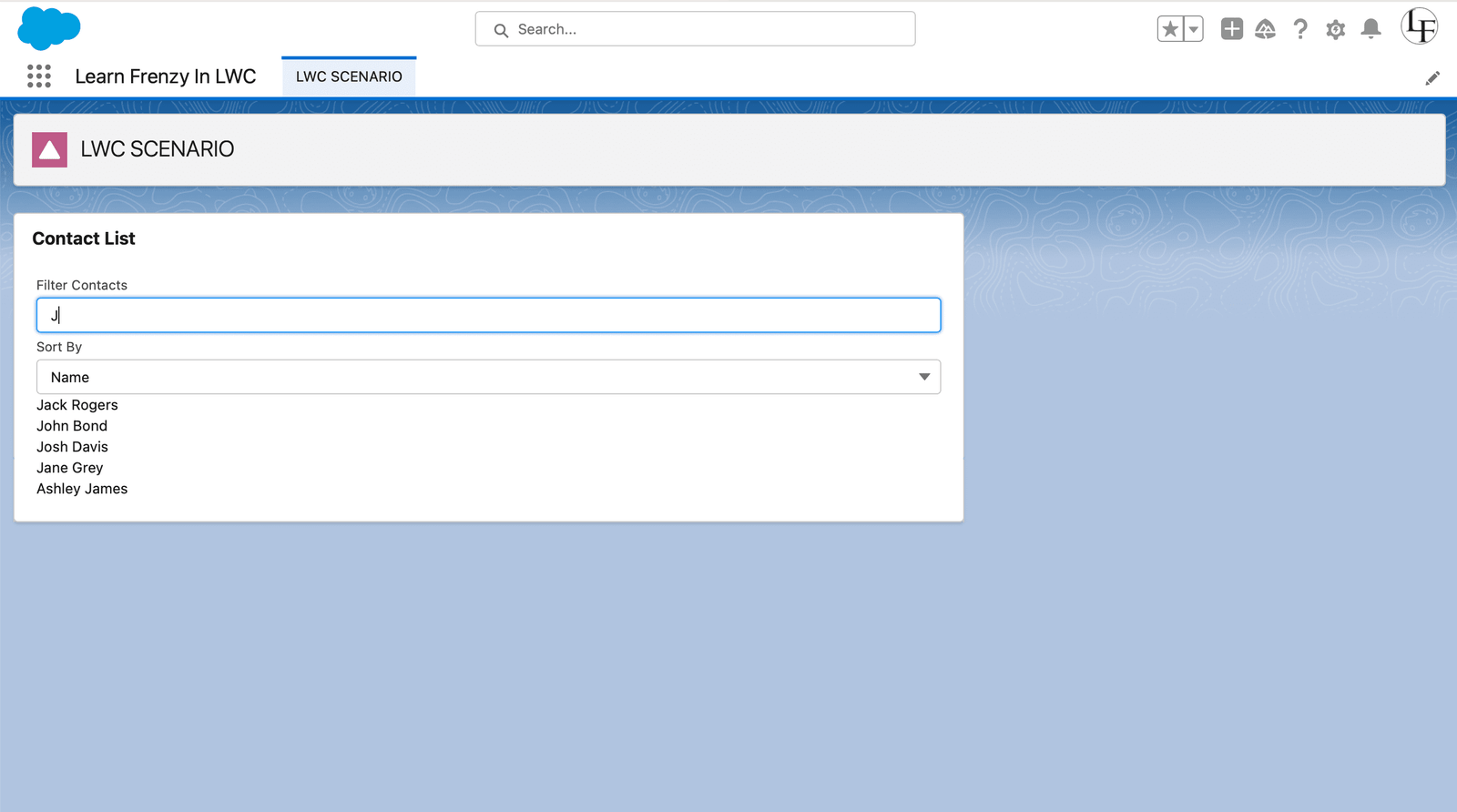
With this implementation, the Lightning Web Component will display a list of Contacts, allow users to filter by name, and sort the Contacts by name or email. This showcases my ability to work with Apex integration, user interface design, and JavaScript logic to create a functional and user-friendly component.
MIND IT !
During the interview for the scenario "Build a Lightning Web Component that displays a list of Contacts and allows users to filter and sort them," the interviewer will assess various aspects of your skills and understanding. Here's what they might check:
1. Component Structure:
• Verify if you can create a Lightning Web Component with the correct files (HTML, JS, CSS) and naming conventions.
• Ensure you've correctly defined the component's attributes, properties, and methods.
2. User Interface Design:
• Check if the user interface is visually appealing and user-friendly.
• Verify that the input elements (like `lightning-input` and `lightning-combobox`) are appropriately used for filtering and sorting.
3. Data Interaction and Display:
• Ensure that the `@wire` decorator is correctly used to fetch and display Contact data from Salesforce.
• Check if the Contacts are displayed in the UI as expected using the `template for:each` directive.
4. Filtering Functionality:
• Verify that the filter functionality is implemented properly and filters Contacts based on user input.
• Check if the filtered Contacts are updated and displayed dynamically as the user types.
5. Sorting Functionality:
• Check if the sorting functionality works correctly when the user selects a sorting option from the combobox.
• Verify that the Contacts are sorted based on the selected field (Name or Email).
6. Apex Integration:
• Ensure that the Apex class (`ContactController`) is correctly defined and annotated with `@AuraEnabled(cacheable=true)`.
• Verify that the Apex method retrieves Contact records and returns them to the Lightning Web Component.
7. JavaScript Logic:
• Check if the JavaScript logic handles filter and sort changes properly.
• Verify that the filtered Contacts are updated without making additional server calls.
8. Code Quality:
• Assess if the code is well-structured, follows best practices, and is easy to understand.
• Check for error handling and proper use of variables and functions.
9. Styling and CSS:
• Ensure that the styling is consistent with Salesforce's Lightning Design System (SLDS).
• Verify that the CSS class names are meaningful and used appropriately.
10. Communication:
• Observe how you explain your code and design choices.
• Check if you can clearly articulate the purpose and functionality of each part of the component.
11. Problem-Solving:
• Check how you handle challenges or unexpected scenarios during the interview.
• Assess your ability to debug issues and make adjustments to the code.
In essence, the interviewer aims to evaluate your skills in Lightning Web Component development, Apex integration, UI design, user interaction, and problem-solving. They also assess your ability to work with data, understand user requirements, and translate them into functional components that adhere to Salesforce's development standards.
LWC Scenario 8 : Pagination and Lazy Loading
Question: Implement pagination in a Lightning Web Component that displays a large list of records and loads more data as users scroll.
Answer:
Scenario Description: You need to create a Lightning Web Component (LWC) that displays a large list of records and implements pagination. Users should be able to load more records by clicking a "Load More" button. This approach optimizes the performance by fetching data progressively and enhances the user experience.
Candidate's Response:
Step 1: Create Lightning Web Component
Create a new Lightning Web Component named "PaginatedRecordList."
Step 2: Design the User Interface (paginatedRecordList.html)
<div class="record-list" onscroll={loadMoreData}> <template for:each={visibleRecords} for:item="record">{record.Name}
</template>
Explanation:
• `<template>`: This is an HTML template tag used in Lightning Web Components to define the structure of the component's markup.
• `<lightning-card>`: This is a standard Lightning component that provides a card-like container with a title. In this case, the title is set to "Paginated Record List."
• `<div class="slds-m-around_medium">`: This `<div>` element has a CSS class applied to it for styling purposes, creating some margin around its content.
• `<div class="record-list" onscroll={loadMoreData}>`: This `<div>` with the class "record-list" has an `onscroll` event listener set to call the "loadMoreData" function when the user scrolls within it. This suggests that this component is designed for displaying a list of records that can be loaded as the user scrolls.
• `<template for:each={visibleRecords} for:item="record">`: This is an iteration template in Lightning Web Components. It iterates over an array or iterable named "visibleRecords" and assigns each item to a variable named "record" within the loop.
• `<p key={record.Id}>{record.Name}</p>`: Inside the loop, this code creates a `<p>` element for each record in the "visibleRecords" array. It displays the "Name" field of each record. The "key" attribute is used for identifying each element uniquely in the loop, typically based on a unique identifier like the "Id" field.
• `<div class="button-section">`: Another `<div>` element with a CSS class applied for styling purposes.
• `<lightning-button label="Load More" onclick={handleLoadMore} disabled={disableLoadMore}></lightning-button>`: This is a Lightning button component with the label "Load More." It has an `onclick` event handler set to call the "handleLoadMore" function when clicked. The "disabled" attribute is bound to the "disableLoadMore" variable, which suggests that the button can be disabled based on some condition.
Step 3: Implement JavaScript Logic (paginatedRecordList.js)
// JS File - paginatedRecordList.js import { LightningElement, track } from 'lwc'; import getRecords from '@salesforce/apex/RecordController.getRecords'; const PAGE_SIZE = 10; export default class PaginatedRecordList extends LightningElement { @track records = []; @track visibleRecords = []; @track disableLoadMore = false; currentPage = 1; connectedCallback() { this.loadRecords(); } loadRecords() { getRecords({ pageSize: PAGE_SIZE, pageNumber: this.currentPage }) .then(result => { this.records = result; this.loadMoreData(); }) .catch(error => { console.error('Error loading records', error); }); } loadMoreData() { const startIndex = (this.currentPage - 1) * PAGE_SIZE; this.visibleRecords = this.records.slice(0, startIndex + PAGE_SIZE); this.disableLoadMore = startIndex + PAGE_SIZE >= this.records.length; } handleLoadMore() { this.currentPage++; this.loadMoreData(); } }
Explanation:
• The JavaScript code defines the `PaginatedRecordList` LWC component.
• The `@track` decorator is used to track the `records`, `visibleRecords`, and `disableLoadMore` properties.
• The `connectedCallback` lifecycle hook loads the initial records when the component is connected to the DOM.
• The `loadRecords` function calls the `getRecords` Apex method to fetch the records for the current page.
• The `loadMoreData` function updates the `visibleRecords` array and disables the "Load More" button when there are no more records to load.
• The `handleLoadMore` function increments the `currentPage` and loads more data when the "Load More" button is clicked.
Step 4: Create Apex Controller (RecordController.cls)
public with sharing class RecordController { @AuraEnabled public static List<Contact> getRecords(Integer pageSize, Integer pageNumber) { return [SELECT Id, Name FROM Contact LIMIT :pageSize OFFSET :pageSize * (pageNumber - 1)]; } }
Step 5: Add Styling (paginatedRecordList.css)
Lastly, I'd apply some CSS to style the component:
/* CSS File - paginatedRecordList.css */ .slds-m-around_medium { margin: 20px; }
Execution and Result:
1. Add the "PaginatedRecordList" component to a Lightning App Page in Salesforce.
2. The component will load the first page of records automatically.
3. Click the "Load More" button to fetch and display the next page of records.
4. The button will be disabled when all records are displayed.
Output:
By following this example, you've implemented pagination in a Lightning Web Component to efficiently display a large list of records. Users can load more records as needed, enhancing the performance and user experience. This scenario demonstrates how LWC can be leveraged to create dynamic and responsive interfaces for handling large data sets.
LWC Scenario 9 : Dependent Picklists
Question: Develop a Lightning Web Component that displays dependent picklists based on the selected value of a controlling picklist.
Answer:
Candidate's Response:
Step 1: Create Lightning Web Component
First, I would create a new Lightning Web Component named "DependentPicklists".
Step 2: Write the HTML Markup (dependentPicklists.html)
<lightning-combobox label="Controlling Picklist" name="controllingPicklist" value={selectedControllingValue} options={controllingOptions} onchange={handleControllingChange} ></lightning-combobox> <lightning-combobox label="Dependent Picklist" name="dependentPicklist" value={selectedDependentValue} options={dependentOptions} ></lightning-combobox>
In this HTML markup:
• We're using two `lightning-combobox` components. One for the controlling picklist and one for the dependent picklist.
• The controlling picklist is used to select a value that will determine the options available in the dependent picklist.
Step 3: Write the JavaScript Logic (dependentPicklists.js)
// JS File - dependentPicklists.js import { LightningElement, track } from 'lwc'; export default class DependentPicklists extends LightningElement { @track selectedControllingValue = ''; @track selectedDependentValue = ''; // Options for controlling and dependent picklists controllingOptions = [ { label: 'Option 1', value: 'Option1' }, { label: 'Option 2', value: 'Option2' } ]; handleControllingChange(event) { this.selectedControllingValue = event.detail.value; if (this.selectedControllingValue === 'Option1') { this.dependentOptions = [ { label: 'A', value: 'A' }, { label: 'B', value: 'B' } ]; } else if (this.selectedControllingValue === 'Option2') { this.dependentOptions = [ { label: 'X', value: 'X' }, { label: 'Y', value: 'Y' } ]; } else { this.dependentOptions = []; // Clear dependent options } } }
In this JavaScript logic:
• We've added two tracked properties, `selectedControllingValue` and `selectedDependentValue`, to keep track of the selected values in the picklists.
• We're using a simple set of options for both controlling and dependent picklists.
• In the `handleControllingChange` method, we update `selectedControllingValue` when the controlling picklist value changes. We also clear the dependent picklist value (`selectedDependentValue`) when the controlling picklist value changes.
MIND IT !
Here's an explanation of the code:
1. Import Statements:
• `import { LightningElement, track } from 'lwc';`: This code imports necessary modules from the LWC framework. `LightningElement` is the base class for LWC, and `track is used to mark properties as reactive, meaning their changes will trigger re-renders in the UI.
2. Class Declaration:
• `export default class DependentPicklists extends LightningElement { ... }`: This defines a new LWC class named `DependentPicklists`. This class extends `LightningElement`, making it a Lightning Web Component.
3. Tracked Properties:
• `@track selectedControllingValue = '';`: This defines a tracked property named `selectedControllingValue` to store the selected value from the controlling picklist.
• `@track selectedDependentValue = '';`: This defines a tracked property named `selectedDependentValue` to store the selected value from the dependent picklist.
4. Controlling Picklist Options:
• `controllingOptions = [ ... ];`: This defines an array named `controllingOptions` that holds the options for the controlling picklist. Each option is represented as an object with `label` and `value` properties.
5. Handle Controlling Change:
• `handleControllingChange(event) { ... }`: This function is called when the value in the controlling picklist changes.
• `this.selectedControllingValue = event.detail.value;`: It updates the `selectedControllingValue` property with the newly selected value from the controlling picklist.
6. Dependent Picklist Options:
• Inside the `handleControllingChange` function, there are conditional statements to determine the options for the dependent picklist based on the selected value in the controlling picklist.
• If 'Option1' is selected, it sets `this.dependentOptions` to an array containing options 'A' and 'B'.
• If 'Option2' is selected, it sets `this.dependentOptions` to an array containing options 'X' and 'Y'.
• If neither 'Option1' nor 'Option2' is selected, it clears `this.dependentOptions` by assigning an empty array.
Step 4: Write the meta.xml (dependentPicklists.js-meta.xml)
58.0 true lightning__AppPage lightning__RecordPage lightning__HomePage
Step 5: Use the Component
Place the "DependentPicklists" component on a Lightning App Page, Record Page, or within another Lightning component where you want to display the dependent picklists.
Output:
In summary, this Lightning Web Component displays dependent picklists based on the selected value of a controlling picklist. It uses hard-coded values for demonstration purposes, but in a real scenario, you would typically fetch picklist values dynamically from Salesforce objects or other data sources.
Extra Knowledge !
Let's create another example of a Lightning Web Component (LWC) that displays dependent picklists based on the selected value of a controlling picklist. In this example, we'll use two custom fields: "Controlling_Picklist__c" and "Dependent_Picklist__c" on the Account object.
Step 1: Create a New Lightning Web Component
Create a new Lightning Web Component named "DependentPicklists".
Step 2: Write the HTML Markup (dependentPicklists.html)
<lightning-combobox label="Controlling Picklist" name="controllingPicklist" value={selectedControllingValue} options={controllingPicklistOptions} onchange={handleControllingPicklistChange} ></lightning-combobox> <lightning-combobox label="Dependent Picklist" name="dependentPicklist" value={selectedDependentValue} options={dependentPicklistOptions} disabled={dependentPicklistDisabled} ></lightning-combobox>
Step 3: Write the JavaScript Logic (dependentPicklists.js)
import { LightningElement, track, wire } from 'lwc'; import { getObjectInfo, getPicklistValues } from 'lightning/uiObjectInfoApi'; import ACCOUNT_OBJECT from '@salesforce/schema/Account'; import CONTROLLING_FIELD from '@salesforce/schema/Account.Controlling_Picklist__c'; import DEPENDENT_FIELD from '@salesforce/schema/Account.Dependent_Picklist__c'; export default class DependentPicklists extends LightningElement { @track selectedControllingValue = ''; @track selectedDependentValue = ''; @track controllingPicklistOptions = []; @track dependentPicklistOptions = []; @track dependentPicklistDisabled = true; @wire(getObjectInfo, { objectApiName: ACCOUNT_OBJECT }) accountObjectInfo; @wire(getPicklistValues, { recordTypeId: '$accountObjectInfo.data.defaultRecordTypeId', fieldApiName: CONTROLLING_FIELD }) wiredControllingPicklist({ data, error }) { if (data) { this.controllingPicklistOptions = data.values.map(option => ({ label: option.label, value: option.value })); } else if (error) { // Handle error } } handleControllingPicklistChange(event) { this.selectedControllingValue = event.detail.value; this.dependentPicklistOptions = []; this.dependentPicklistDisabled = true; this.loadDependentPicklistOptions(); } loadDependentPicklistOptions() { // Fetch dependent picklist options based on the selected controlling value // You can use Apex, SOQL, or any other suitable method // Assign the dependent options to this.dependentPicklistOptions } }
In this example:
• We use the `lightning-combobox` component for both the controlling and dependent picklists.
• We use the `getObjectInfo` and `getPicklistValues` wire adapters to dynamically fetch the controlling picklist options based on the Account object's schema.
• The `handleControllingPicklistChange` function is called when the controlling picklist value changes. It resets the dependent picklist and triggers the loading of dependent picklist options using the `loadDependentPicklistOptions` function.
• In the `loadDependentPicklistOptions` function, you would typically fetch dependent picklist options based on the selected controlling value. This could involve using Apex, SOQL queries, or any other data retrieval method.
In this example, we've focused on creating a foundation for displaying dependent picklists. You would need to implement the data retrieval and loading of dependent picklist options according to your specific use case and Salesforce data structure.
(1) Comments
Got a good knowledge with this article, Thanks!!